In the last article, I summarized the process of enabling and using GPIO pins on the Okdo E1 by configuring the MCU in the MCUXpresso IDE. This time, I’ll enable pin interrupts that allow an application to react to various events.
Before you begin
Make sure to go over the steps in the previous tutorial. This way you can make sure that your project is properly configured and set up to work with the Okdo E1 board. It furthermore explains how to open the tools you’ll need to utilize in this tutorial.
Loading additional drivers
In the project we created in the last tutorial, the GPIO driver was already added to the project by default. To use interrupts, we’ll, however, need to add an additional driver. To do that, click the small brown package icon in the project explorer tab in the main development view of the IDE:

If the package icon is greyed out, make sure that your project is highlighted in the explorer. Furthermore, make sure that no other project is selected. Otherwise, you’ll edit the properties of that project instead of the current one.
Doing this will open up the following pop-up window where you can search for drivers. Click the ‘Drivers’ tab and search for the pint module. Once you found it, tick the checkbox next to it and click the ‘OK’ button:

After that, search for the ‘inputmux’ driver and add it to your project as well. Once you’re done, click the ‘OK’ button. The IDE will reload the project and you should be ready to use interrupts now!
Setting up pin interrupts in the MCUXpresso IDE
The process of configuring pins to react to interrupts is pretty much the same as for setting up GPIO pins. Therefore, I’ll speed through the process. Please refer to the previous tutorial for a more detailed explanation.
Anyway, navigate to the ‘Pins’ tool of the IDE and search for the pin named ‘PIO1_9’. If you remember the pinout of the Okdo E1, then you’ll notice that this pin is connected to one of the user buttons on the side of the board:

Once you found the pin (which is pin #10 by the way) route the PINT signal channel 0 to PIO1_9:

This will route the pin to channel zero of the PINT module. Once you’re done, click the ‘Update Code’ button and navigate back to your code.
Handling interrupts
To make interrupts work in your code, you’ll first have to include the following header file:
#include "fsl_pint.h"
Then, initialize the PINT module in your main method. You’ll only have to do this once:
int main(void)
{
/* BOARD INITIALIZATION */
// Initialize the PINT module
PINT_Init(PINT);
/* OTHER STUFF */
}
Next, configure the interrupts for each channel. The interrupt config method takes a few parameters. The first one describes the base address of the PINT peripheral. The next one describes the channel (remember: we chose channel 0 in the pins tool earlier). The next parameter describes what detection logic you want to use. This lets you choose whether you want the callback to be called on falling edges, rising edges, or both. The last parameter contains a custom callback function that will be called on every button press:
// Make PINT channel 0 react to rising edges
PINT_PinInterruptConfig(PINT, kPINT_PinInt0, kPINT_PinIntEnableRiseEdge, board_button_pressed);
These steps will configure the interrupts. You’ll only have to call these functions once. The interrupt config, however, will have to be called for each PINT channel individually.
Next, you’ll have to define a function that will be called when the button is pressed. For now, let’s just accept the fact that it looks like this:
void board_button_pressed(pint_pin_int_t pintr, uint32_t pmatch_status)
{
printf("Button pressed!\n");
}
The first parameter contains a number that describes which PINT channel caused the interrupt, the second one contains the pmatch_status that you can use for pattern matching (Refer to the API for this topic).
If you now compile the code and upload it to your board, you’ll see that nothing happens when you press the button. That is, because you’ll also have to enable the callback that we configured earlier:
// Enable callbacks for PINT0
PINT_EnableCallbackByIndex(PINT, kPINT_PinInt0);
I wanted to mention this separately because you can also remove callbacks at any time and re-enable them later on. This is especially useful if you only want to detect the first falling/rising edge (for example to determine the start of a transmission sequence), and have the rest of the decoding handled by another callback. Anyway, you can remove callbacks with the following function:
PINT_DisableCallbackByIndex(PINT, kPINT_PinInt0);
I think the parameters of these two functions are pretty self-explanatory. The first one describes the base address, the second one lets you select a PINT channel. Note that there are also variants of this function that don’t distinguish between the individual PINT channels:
PINT_EnableCallback(PINT);
PINT_DisableCallback(PINT);
Calling these will enable/disable all PINT channels at once.
To de-initialize the PINT module, call the following function:
PINT_Deinit(PINT);
Example code
Below is a complete example that sets up PINT 0 and outputs a short message whenever a rising edge is detected on PIO1_9:
#include <stdio.h>
#include "board.h"
#include "peripherals.h"
#include "pin_mux.h"
#include "clock_config.h"
#include "LPC55S69_cm33_core0.h"
#include "fsl_debug_console.h"
#include "fsl_pint.h"
void board_button_pressed(pint_pin_int_t pintr, uint32_t pmatch_status)
{
printf("Button pressed!\n");
}
int main(void)
{
// Init board hardware
BOARD_InitBootPins();
BOARD_InitBootClocks();
BOARD_InitBootPeripherals();
// Init FSL debug console
BOARD_InitDebugConsole();
// Initialize the PINT module
PINT_Init(PINT);
// Make PINT channel 0 react to rising edges
PINT_PinInterruptConfig(PINT, kPINT_PinInt0, kPINT_PinIntEnableRiseEdge, board_button_pressed);
// Enable callbacks for PINT0
PINT_EnableCallback(PINT);
PRINTF("Hello World\n");
while(true)
{
__asm volatile ("nop");
}
return 0;
}
Note that this code will only work if you configure the pins appropriately and load the necessary drivers as described in this article.
Image sources
[Title image] Original image courtesy of allaboutcircuits.com
[Figure 7] Okdo E1 pinout diagram – okdo.com
Sources
PINT module API documentation – mcuxpresso.nxp.com
Table of contents
How to use the GPIO pins of the LPC55S69
How to use interrupts on the LPC55S69 (You are here)
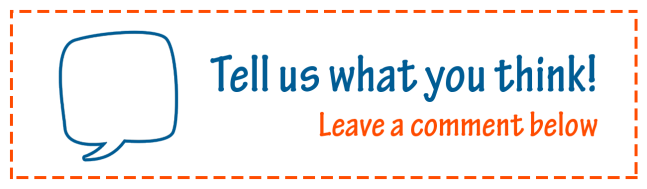
One thought on “How to use interrupts on the LPC55S69 powered Okdo E1”